Introduction
Web Services are a very general model for building applications and can be implemented for any operation system that supports communication over the Internet. Web Services use the best of component-based development and the Web. Component-base object models like Distributed Component Object Model (DCOM), Remote Method Invocation (RMI), and Internet Inter-Orb Protocol (IIOP) have been around for some time. Unfortunately all these models depend on an object-model-specific protocol. Web Services extend these models a bit further to communicate with the Simple Object Access Protocol (SOAP) and Extensible Markup Language (XML) to eradicate the object-model-specific protocol barrier (see Figure 1). Web Services basically uses Hypertext Transfer Protocol (HTTP) and SOAP to make business data available on the Web. It exposes the business objects (COM objects, Java Beans, etc.) to SOAP calls over HTTP and executes remote function calls. The Web Service consumers are able to invoke method calls on remote objects by using SOAP and HTTP over the Web.
How is the user at Location A aware of the semantics of the Web Service at Location B? This question is answered by conforming to a common standard. Service Description Language (SDL), SOAP Contract Language (SCL) and Network Accessible Specification Language (NASSL) are some XML-like languages built for this purpose. However, IBM and Microsoft recently agreed on the Web Service Description Language (WSDL) as the Web Service standard. The structure of the Web Service components is exposed using this Web Service Description Language. WSDL 1.1 is a XML document describing the attributes and interfaces of the Web Service. The new specification is available at msdn.microsoft.com/xml/general/wsdl.asp.
Microsoft .NET marketing has created a huge hype about its Web Services. This is the first of two articles on Web Services. Here we will create a .NET Web Service using C#. We will look closely at the Discovery protocol, UDDI, and the future of the Web Services. In the next article, we will concentrate on consuming existing Web Services on multiple platforms (i.e., Web, WAP-enabled mobile phones, and windows applications).
Why do we need Web Services?
After buying something over the Internet, you may have wondered about the delivery status. Calling the delivery company consumes your time, and it's also not a value-added activity for the delivery company. To eliminate this scenario the delivery company needs to expose the delivery information without compromising its security. Enterprise security architecture can be very sophisticated. What if we can just use port 80 (the Web server port) and expose the information through the Web server? Still, we have to build a whole new Web application to extract data from the core business applications. This will cost the delivery company money. All the company wants is to expose the delivery status and concentrate on its core business. This is where Web Services come in.
What is a Web Service?
Figure 1. SOAP calls are remote function calls that invoke method executions on Web Service components at Location B. The output is rendered as XML and passed back to the user at Location A.
The task ahead
The best way to learn about Web Services is to create one. We all are familiar with stock quote services. The NASDAQ, Dow Jones, and Australian Stock Exchange are famous examples. All of them provide an interface to enter a company code and receive the latest stock price. We will try to replicate the same functionality. The input parameters for our securities Web service will be a company code. The Web service will extract the price feed by executing middle-tier business logic functions. The business logic functions are kept to a bare minimum to concentrate on the Web service features.
Tools to create a Web Service
The core software component to implement this application will be MS .NET Framework SDK, which is currently in beta. You can download a version from Microsoft. I used Windows 2000 Advance Server on a Pentium III with 300 MB of RAM. The preferred Integration Development Environment (IDE) to create Web Services is Visual Studio .NET. However, you can easily use any text editor (WordPad, Notepad, Visual Studio 6.0) to create a Web Service file. I assume you are familiar with the following concepts:
Creating a Web Service
We are going to use C# to create a Web Service called "SecurityWebService." A Web Service file will have an .ASMX file extension. (as opposed to an .ASPX file extension of a ASP.NET file). The first line of the file will look like Dot-net Web Services are intelligent enough to cast basic data types. Therefore, if we return "int," "float," or "string" data types, it can convert them to standard XML output. Unfortunately, in most cases we need get a collection of data regarding a single entity. Let's take an example. Our SecurityWebService stock quotes service requires the user to enter a company code, and it will deliver the full company name and the current stock price. Therefore, we have three pieces of information for a single company: We can use the business logic in this function to get the newest stock price quote. For the purpose of this article I have added some text to the company code to create the company name. The price value is generated using a random number generator. We may save this file as "SampleService.asmx" under an Internet Information Service (IIS)-controlled directory. I have saved it under a virtual directory called "/work/aspx." I'll bring it up on a Web browser. This is a Web page rendered by the .NET Framework. We did not create this page. (The page is generated automatically by the system. I did not write any code to render it on the browser. This graphic is a by-product of the previous code.) This ready-to-use functionality is quite adequate for a simple Web Service. The presentation of this page can be changed very easily by using ASP.NET pagelets and config.web files. A very good example can be found at
http://www.ibuyspy.com/store/InstantOrder.asmx. Notice a link to "SDL Contract." (Even if we are using WSDL, .NET Beta still refers to SDL. Hopefully this will be rectified in the next version). This is the description of the Web Service to create a proxy object. (I will explain this in the next article.) This basically gives an overview of the Web Service and it's public interface. If you look closely, you will only see the "Web-only" methods being illustrated. All the private functions and attributes are not described in the SDL contract.
This line will instruct the compiler to run on Web Service mode and the name of the C# class. We also need to access the Web Service namespace. It is also a good practice to add a reference to the System namespace.
<%@ WebService Language="C#" class="SecurityWebService" %>
The SecurityWebService class should inherit the functionality of the Web Services class. Therefore, we put the following line of code:
using System;
using System.Web.Services;
Now we can use our object-oriented programming skills to build a class. C# classes are very similar to C++ or Java classes. It will be a walk in the park to create a C# class for anyone with either language-coding skills.
public class SecurityWebService : WebService
We need to extract all this data when we are referring to a single stock quote. There are several ways of doing this. The best way could be to bundle them in an enumerated data type. We can use "structs" in C# to do this, which is very similar to C++ structs.
Now we have all the building blocks to create our Web Service. Therefore, our code will look like.
public struct SecurityInfo
{
public string Code;
public string CompanyName;
public double Price;
}
Remember, this Web Service can be accessed through HTTP for any use. We may be referring to sensitive business data in the code and wouldn't want it to fall into the wrong hands. The solution is to protect the business logic function and only have access to the presentation functions. This is achieved by using the keyword "[Web Method]" in C#. Let's look at the function headers of our code.
<%@ WebService Language="C#" class="SecurityWebService" %>
using System;
using System.Web.Services;
public struct SecurityInfo
{
public string Code;
public string CompanyName;
public double Price;
}
public class SecurityWebService : WebService
{
private SecurityInfo Security;
public SecurityWebService()
{
Security.Code = "";
Security.CompanyName = "";
Security.Price = 0;
}
private void AssignValues(string Code)
{
// This is where you use your business components.
// Method calls on Business components are used to populate the data.
// For demonstration purposes, I will add a string to the Code and
// use a random number generator to create the price feed.
Security.Code = Code;
Security.CompanyName = Code + " Pty Ltd";
Random RandomNumber = new System.Random();
Security.Price = double.Parse(new System.Random(RandomNumber.Next(1,10)).NextDouble().ToString("##.##"));
}
[WebMethod(Description="This method call will get the company name and the price for a given security code.",EnableSession=false)]
public SecurityInfo GetSecurityInfo(string Code)
{
AssignValues(Code);
SecurityInfo SecurityDetails = new SecurityInfo();
SecurityDetails.Code = Security.Code;
SecurityDetails.CompanyName = Security.CompanyName;
SecurityDetails.Price = Security.Price;
return SecurityDetails;
}
}
This function is exposed to the public. The "description" tag can be used to describe the Web Service functionality. Since we will not be storing any session data, we will disable the session state.
[WebMethod(Description="This......",EnableSession=false)]
public SecurityInfo GetSecurityInfo(string Code)
This is a business logic function that should not be publicly available. We do not want our sensitive business information publicly available on the Web. (Note:- Even if you change the "private" keyword to "public," it will still not be publicly available. You guessed it, the keyword "[Web Method]" is not used.)
private void AssignValues(string Code)
How do we use a Web Service?
You can also call the Web Service directly using the HTTP GET method. In this case we will not be going through the above Web page and clicking the Invoke button. The syntax for directly calling the Web Service using HTTP GET is Now we can use this Web Service. Let's enter some values to get a bogus price feed. By clicking the Invoke button a new window will appear with the following XML document This is how the Web Service releases information. We need to write clients to extract the information from the XML document. Theses clients could be http://server/webServiceName.asmx/functionName?parameter=parameterValue Therefore, the call for our Web Service will be http://localhost/work/aspx/SampleService.asmx/GetSecurityInfo?Code=IBM This will produce the same result as clicking the Invoke button. Now we know how to create a Web Service and use it. But the work is half done. How will our clients find our Web Service? Is there any way to search for our Web Service on the Internet? Is there a Web crawler or a Yahoo search engine for Web Services? In order to answer these questions we need to create a "discovery" file for our Web Service.
Creating a Discovery file
Web Service discovery is the process of locating and interrogating Web Service descriptions, which is a preliminary step for accessing a Web Service. It is through the discovery process that Web Service clients learn that a Web Service exists, what its capabilities are, and how to properly interact with it. Discovery file is a XML document with a .DISCO extension. It is not compulsory to create a discovery file for each Web Service. Here is a sample discovery file for our securities Web Service. There have been a lot of Web Services written by developers. www.xmethods.com is one of the sites that has an index of Web Services. Some developers are building WSDL search engines to find Web Services on the Web.
We can name this file "SampleService.disco" and save it to the same directory as the Web Service. If we are creating any other Web Services under the "/work/aspx" directory, it is wise to enable "dynamic discovery." Dynamic discovery will scan for all the *.DISCO files in all the subdirectories of "/work/aspx" automatically.
An example of an active discovery file can be found at http://services3.xmethods.net/dotnet/default.disco. By analyzing the discovery file we can find where the Web Services reside in the system. Unfortunately both these methods require you to know the exact URL of the discovery file. If we cannot find the discovery file, we will not be able to locate the Web Services. Universal Description, Discovery, and Integration (UDDI) describes mechanisms to advertise existing Web Services. This technology is still at the infant stage. UDDI is an open, Internet-based specification designed to be the building block that will enable businesses to quickly, easily, and dynamically find and transact business with one another using their preferred applications. A reference site for UDDI is http://uddi.microsoft.com.
Deploying a Web Service
Deploying the Web Services from development to staging or production is very simple. Similar to ASP.NET applications, just copy the .ASMX file and the .DISCO files to the appropriate directories, and you are in business. The future of the Web Services The future looks bright for the Web Service technology. Microsoft is not alone in the race for Web Service technology. Sun and IBM are very interested. There are SOAP toolkits available for Apache and Java Web servers. I believe Web Services needs a bit of work, especially the Web Service discovery process. It is still very primitive. On a positive note, Web Services have the potential to introduce new concepts to the Web. One I refer to as "pay per view" architecture. Similar to pay-TV, we can build Web sites that can generate revenue for each request a user sends (as opposed to a flat, monthly subscription). In order to get some data, we can sometimes pay a small fee. Commercially this could be handy for a lot of people.
Examples
On a very optimistic note, Web Services can be described as the "plug and play" building blocks of enterprise Business to Business (B2B) Web solutions.
And the list goes on ...

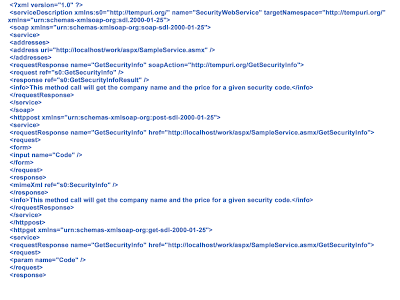
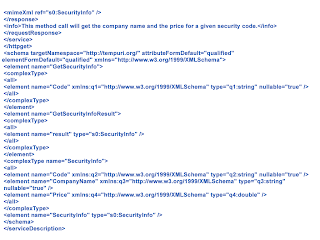